Using the VPS Coverage API
The VPS Coverage API is used to discover VPS coverage areas and understand how best to localize with VPS localization targets.
You can use the VPS Coverage API to find VPS coverage areas around a given geographic location. The ARDK backend will provide a list of geographic coverage areas where a user can use VPS to localize. A coverage area may contain a set of VPS localization targets powered by VPS-activated Wayspots, which help the user localize within the area. You can use the VPS Coverage API to get details about localization targets, including the target name, target latitude and longitude, and a “hint image” photo of the target.
Use the VPS Coverage API when you need to guide your users to nearby VPS coverage areas, or help your users find and localize VPS targets within a particular area. Helping your users find VPS localization targets will make the actual VPS localization process much smoother for the user. You’d typically want to use this API to guide users to an VPS coverage area and show them what to point their AR-enabled device at before starting VPS localization.
Note
VPS Coverage API only returns information about publicly-accessible VPS coverage areas and localization targets. Currently, a VPS localization target is associated with a VPS-activated Wayspot. VPS Coverage API can’t be used to get information about Private VPS Locations.
Typical User Flow When Using the VPS Coverage API
Before your users can localize with VPS, they need to know information such as where a valid VPS coverage area is, how far they are from the location, and what they should be focusing on with their AR-enabled device when they localize. You can use the following flow to provide this information:
First, determine what VPS coverage areas are available to the player. The VPS Coverage API can provide a list of coverage areas near a specific geographic location and zero or more localization targets within a coverage area. You can display coverage areas and targets (typically in a map-based UI element) to help your users inspect and choose areas and targets that they can visit. Users can also use coverage area information to determine how close they are to the coverage area where they can start to localize.
Next, as your user inspects or chooses particular localization targets, you can make VPS Coverage API calls to get detailed information about the selected targets. Target details, such as the name, GPS location, and “hint image” photo of the target, can be displayed to the user to help them understand what localization targets they’re looking for. You can also use a 3rd party map API to calculate directions for the user on how to get to a selected target location.
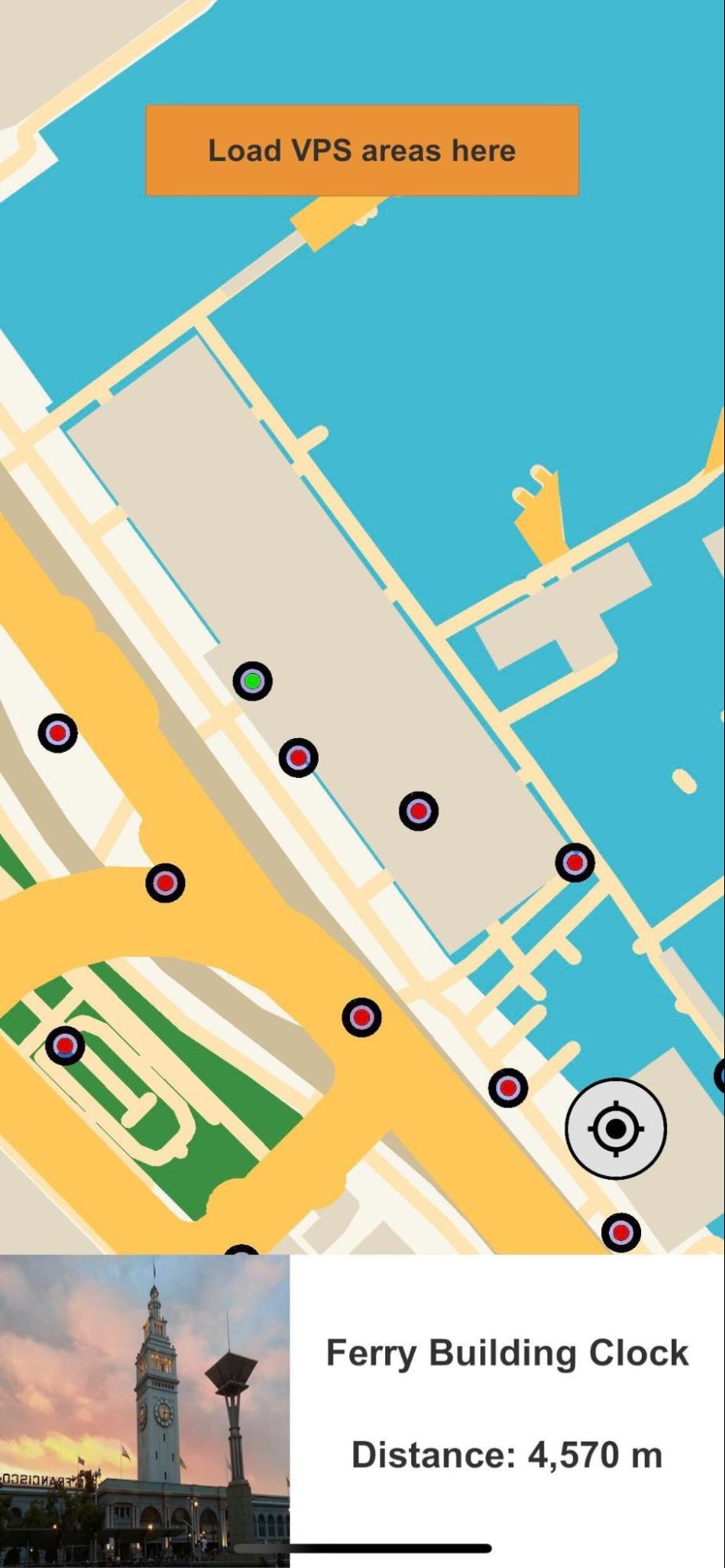
Finally, once the user has selected a localization target and moved within the coverage area for the target, you can instruct them on how to begin the VPS localization process, as described in Localizing with VPS.
Note
Use of features such as the VPS Coverage API involves the collection of personal information from your end user. For more information, see the Lightship ARDK Data Privacy FAQ.
Enable Location Permissions
Because the VPS Coverage API uses device location information, you’ll need to make sure the user has enabled location permissions on their device. See Permissions for details on requesting device permissions.
Discover VPS Coverage Areas and Localization Targets
You can use the VPS Coverage API to find VPS coverage areas near a specific geographic location. This location can be based on the current user or device location, or you can specify a latitude & longitude. You may need to provide a specific location if your app uses a map UI and you want to display locations relative to the center of the map as the user pans the map.
To use the VPS Coverage API to find locations near the current device location, you’ll need to do the following:
Create an ILocationService instance and subscribe to the
OnLocationUpdated
event to get updates for the current device location. If you’re running in the Unity editor, you can useSpoofLocationService
to set a spoofed location.
Note
If you want to use a specific location rather than the device location, you don’t need to use a ILocationService
.
using Niantic.ARDK.LocationService; private ILocationService _locationService; // Default is the Ferry Building in San Francisco private LatLng _spoofLocation = new LatLng(37.79531921750984, -122.39360429639748); // Start is called before the first frame update void Awake() { // ... _locationService = LocationServiceFactory.Create(); #if UNITY_EDITOR var spoofService = (SpoofLocationService) _locationService; // In editor, the specified spoof location will be used. spoofService.SetLocation(_spoofLocation); #endif _locationService.LocationUpdated += OnLocationUpdated; _locationService.Start(); }
Create an ICoverageClient instance used to make VPS Coverage API requests.
using Niantic.ARDK; using Niantic.ARDK.VPSCoverage; using Niantic.ARDK.VirtualStudio.VpsCoverage; private RuntimeEnvironment _coverageClientRuntime = RuntimeEnvironment.Default; private ICoverageClient _coverageClient; private VpsCoverageResponses _mockResponses; void Awake() { // ... // The mockResponses object is a ScriptableObject containing the data that a Mock // implementation of the ICoverageClient will return. This is a required argument for using // the mock client on a mobile device. It is optional in the Unity Editor; the mock client // will simply use the data provided in the ARDK/VirtualStudio/VpsCoverage/VPS Coverage Responses.asset file. _coverageClient = CoverageClientFactory.Create(_coverageClientRuntime, _mockResponses); // ... }
In your
OnLocationUpdated
event handler, useICoverageClient.RequestCoverageAreas()
with the new location info to request nearby locations. In your request you need to provide:
The desired location latitude and longitude
A request radius in meters (up to 2000 meters). VPS Coverage API will return VPS coverage areas that are within this radius of the request location.
You’ll also provide a callback to RequestCoverageAreas()
that will be called when the response is received. If you prefer to use the Task-based async await pattern, you can use RequestCoverageAreasAsync()
. Both RequestCoverageAreas()
and RequestCoverageAreasAsync()
are non-blocking calls.
void OnLocationUpdated(LocationUpdatedArgs args) { _locationService.LocationUpdated -= OnLocationUpdated; _coverageClient.RequestCoverageAreas(args.LocationInfo, _queryRadius, ProcessAreasResult); }
Process the response.
async void ProcessAreasResult(CoverageAreasResult result) { // ... if (result.Status != ResponseStatus.Success) return; foreach (var area in result.Areas) { // Process coverage areas accordingly } }
If the request is successful, the response will contain a list of CoverageAreas near the request location, within the request radius. Each CoverageArea contains:
Zero or more localization target IDs
A coverage area shape. The shape is a simple (no holes) polygon defined by an array of latitude and longitude points in clockwise order.
A localizability quality value, which gives an estimate of how easy it will be for users to localize in that area. A coverage area with a
LocalizabilityQuality
of PRODUCTION means that users should be able to localize in that area under a wide range of conditions. A coverage area with aLocalizabilityQuality
of EXPERIMENTAL means user localizations in that area might not work under all conditions.
Note that:
A coverage region is currently constrained to roughly a 5 meter radius around a localization target. This radius may increase in the future.
If the user has not allowed location permissions, or the device has location permissions disabled, the request will fail. A
LocationService.StatusUpdated
event will be invoked with the specific error (user permission not granted, or system/device level location disabled)
See the VpsCoverageExample and VpsCoverageListExample samples in the ARDK-Examples package for more examples of getting coverage areas.
Get VPS Localization Target Details
Once you’ve obtained a list of localization target IDs, use RequestLocalizationTargets()
or RequestLocalizationTargetsAsync()
to get detailed information about each target. You’ll need an ICoverageClient
instance to make the request, as described in the previous section.
Provide an array of target IDs you want detailed information about. The list of CoverageAreas
returned from a RequestCoverageAreas()
request contains target IDs within the given coverage areas. The following example extracts the list of target IDs from the list of CoverageAreas and passes this to a call to RequestLocalizationTargets()
.
async void ProcessAreasResult(CoverageAreasResult result) { var allTargets = new List<string>(); if (result.Status != ResponseStatus.Success) return; foreach (var area in result.Areas) { allTargets.AddRange(area.LocalizationTargetIdentifiers); } _coverageClient.RequestLocalizationTargets(allTargets.ToArray(), ProcessTargetsResult); }
If the request is successful, you’ll get target details in a dictionary of LocalizationTargets that you can process.
async void ProcessTargetsResult(LocalizationTargetsResult result) { if (result.Status != ResponseStatus.Success) return; if (result.ActivationTargets.Count > 0) { Vector2 imageSize = TargetImage.rectTransform.sizeDelta; LocalizationTarget firstTarget = result.ActivationTargets.FirstOrDefault().Value; firstTarget.DownloadImage((int)imageSize.x, (int)imageSize.y, args => _targetImage.texture = args); // retrieve other LocalizationTarget details and display to user as needed } }
Each LocalizationTarget contains the target ID, a target name, the latitude/longitude of the target, and a “hint image” URL that points to an image of the target. LocalizationTarget
also provides DownloadImage()
and DownloadImageAsync()
convenience methods to download the hint image to a texture. Use the hint image to show your users a picture of what they need to point their device camera at when they need to do VPS localization.
Here’s an example screenshot showing a list of retrieved target details, including hint images:
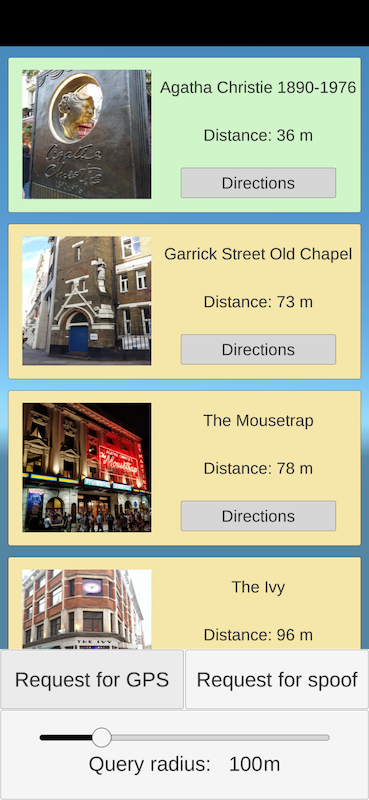
See the VpsCoverageExample and VpsCoverageListExample samples in the ARDK-Examples package for more examples of RequestLocalizationTargets()
requests and how to process the results.
Displaying Locations to the User
How you display coverage area and localization target information to your users will depend on your use case and application design, however one common method is using a map UI element.
You can get started with Lightship Maps for Unity. See How to Integrate the VPS Coverage API with Lightship Maps for more information.