Virtual Studio
Improving the experience of developing with ARDK using Virtual Studio.
Overview
Virtual Studio adds two methods of running AR and networking functionality to improve the experience of developing with ARDK in Unity. Both methods eliminate some steps from the iteration loop required to test code changes in your application, allowing you to develop without sinking time into building and deploying your app to the device.
With the Remote features, your code can run solely in Unity Editor’s Play Mode, allowing for code iteration to happen without re-deploying to a device. Under the hood, ARDK communicates with an instance of the Remote Debugger app to run ARDK code on the mobile device and send relevant data back to Unity.
Note
Remote mode over the internet (not Remote mode over USB) is being deprecated as of ARDK release 2.1.
With the Mock features, all AR and networking functionality is mocked inside the Unity Editor, eliminating the need for a mobile device. Simulated environments and mock multiplayer setups can be used to test your code in various AR and networking scenarios.
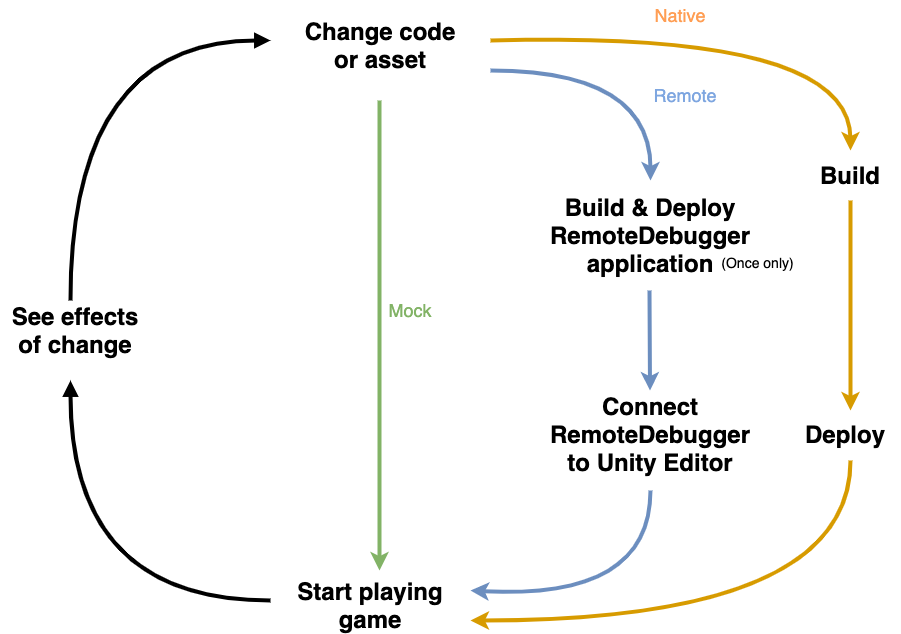
Setting up your code to utilize Virtual Studio
Each of the core ARDK interfaces (IARSession, IMultipeerNetworking, and IARNetworking) can be constructed through their respective factories to run from a live device, through remote, or through mock at runtime, depending on platform conditions.
using Niantic.ARDK.AR.Configuration; // Example code to construct and run an AR session that can be run // through Virtual Studio. There's no difference from creating one that // runs on a mobile device — it's the same code. void CreateAndRunARSession() { var arSession = ARSessionFactory.Create(); arSession.Run(ARWorldTrackingConfigurationFactory.Create()); }
Each factory’s Create
method will create their respective object from the first valid given runtime environment option. If no options are given, then the default order tried is:
Live Device - will succeed if called on a mobile platform (Android or iOS).
Remote - will succeed if the Use Remote checkbox is checked in the Remote Connector window.
Mock - will succeed if called in the Unity Editor.
Platform-Agnostic Input
ARDK provides a cross-platform class called PlatformAgnosticInput that unifies Unity’s mouse and Touch input APIs. Left (or primary) mouse-clicks in the Unity Editor will register the same as touch input on a mobile device. Use this class to be able to play through your application in the Unity Editor as well as on a phone.
Hit-Testing
Hit-tests against planes can be invoked the same whether done on device or through Virtual Studio (the Hit-Testing page explains how to do that). However, all ARHitTestResultType values excluding ARHitTestResultType.ExistingPlaneUsingExtent
are not supported in either Virtual Studio’s Mock or Remote mode.
Live Device Networking in the Unity Editor
Unlike the AR stack, ARDK’s networking stack can run natively on the MacOS platform, meaning it can be used in the Unity Editor and function the same as on Android or iOS devices.
The networking stack cannot be used on Windows platforms. Only MultipeerNetworking instances using the Mock or Remote runtime environments will be successfully created.
using Niantic.ARDK; using Niantic.ARDK.Networking; using System.Text; public bool _useLiveDeviceInEditor; void CreateAndJoinNetworking() { IMultipeerNetworking networking; if (_useLiveDeviceInEditor) networking = MultipeerNetworkingFactory.Create(RuntimeEnvironment.LiveDevice); else networking = MultipeerNetworkingFactory.Create(RuntimeEnvironment.Default); var sessionMetadata = Encoding.UTF8.GetBytes("ExampleSessionName"); networking.Join(sessionMetadata); }
Multiple objects created for different runtime environments, however, cannot exist simultaneously. That is, all the ARSession, MultipeerNetworking, and ARNetworking objects created in the same instance of your application must be for the same RuntimeEnvironment
.